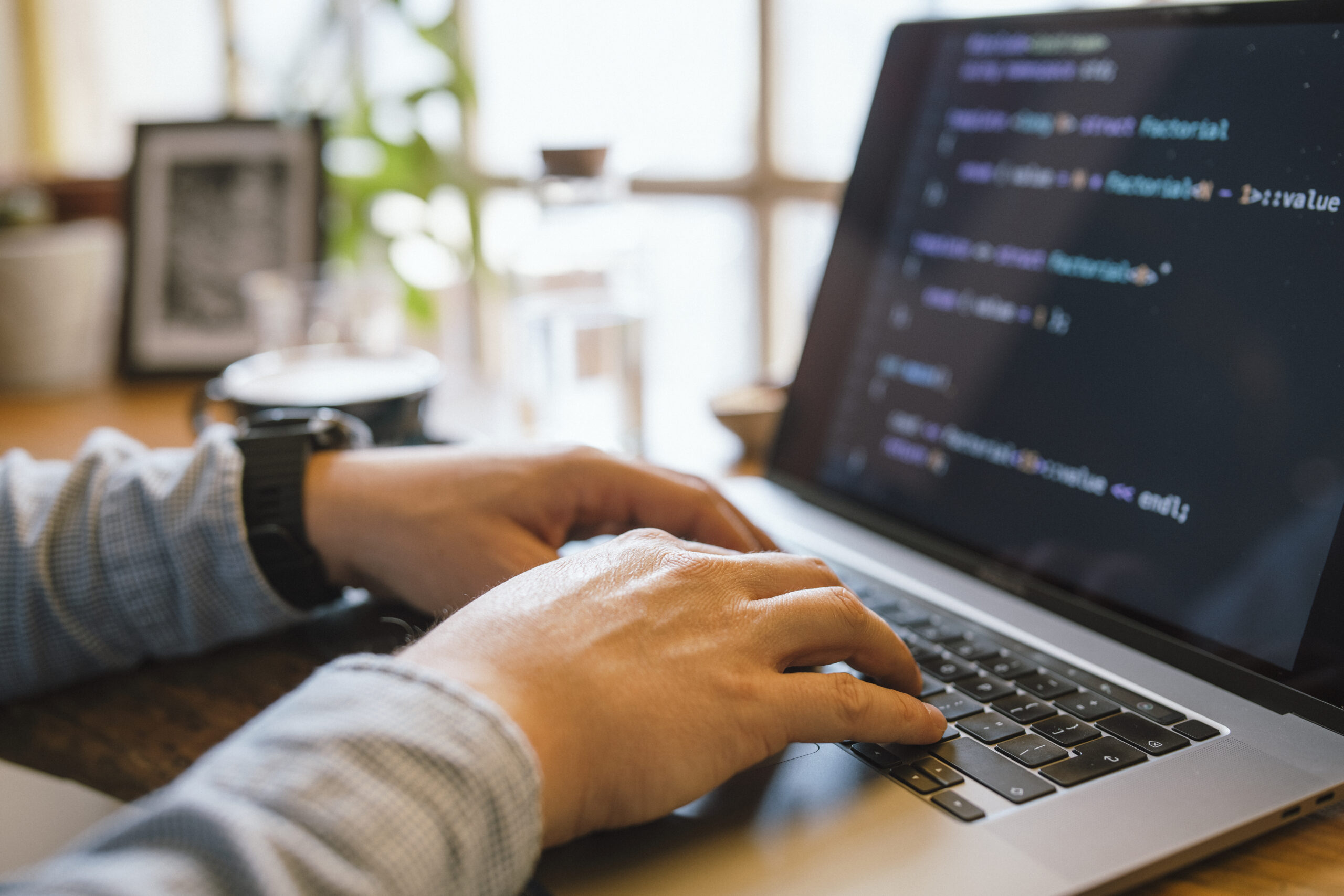
Debugging is One of the more important — still normally overlooked — expertise within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go wrong, and Studying to Believe methodically to solve issues effectively. No matter whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Listed here are several strategies to help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is a single part of enhancement, figuring out the way to interact with it correctly through execution is equally important. Modern-day advancement environments occur Geared up with impressive debugging abilities — but numerous builders only scratch the area of what these equipment can do.
Acquire, by way of example, an Integrated Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and even modify code about the fly. When utilized the right way, they Allow you to notice specifically how your code behaves during execution, that's invaluable for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude developers. They enable you to inspect the DOM, keep track of community requests, view true-time general performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into workable tasks.
For backend or program-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with Variation Manage techniques like Git to be aware of code record, find the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about establishing an personal expertise in your development atmosphere in order that when troubles occur, you’re not dropped at nighttime. The higher you recognize your instruments, the greater time it is possible to commit fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
Probably the most critical — and infrequently missed — ways in productive debugging is reproducing the situation. Ahead of leaping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug becomes a video game of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What steps resulted in The difficulty? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered ample information, endeavor to recreate the issue in your neighborhood setting. This could indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automated exams that replicate the sting cases or condition transitions associated. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, the issue could be natural environment-particular — it would transpire only on certain working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a stage — it’s a attitude. It involves tolerance, observation, and a methodical approach. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination likely fixes safely and securely, and converse far more Plainly using your staff or end users. It turns an summary criticism right into a concrete problem — and that’s exactly where developers thrive.
Read and Understand the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, exactly where it transpired, and from time to time even why it took place — if you know how to interpret them.
Start by looking at the concept cautiously As well as in entire. Numerous builders, particularly when under time tension, glance at the first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the correct root induce. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it point to a certain file and line number? What module or operate triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can drastically quicken your debugging course of action.
Some errors are obscure or generic, As well as in those circumstances, it’s important to examine the context during which the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Sensibly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed efficiently, it provides actual-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic data in the course of advancement, Information for general events (like thriving start out-ups), Alert for prospective problems that don’t break the applying, ERROR for real problems, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your program. Focus on vital functions, state variations, input/output values, and critical final decision factors in your code.
Structure your log messages clearly and continually. Contain context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to track how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. That has a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot concerns, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Like a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently establish and take care of bugs, developers should technique the procedure similar to a detective resolving a mystery. This state of mind aids stop working advanced challenges into workable parts and adhere to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire just as much appropriate data as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and person stories to piece jointly a clear image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What could be producing this actions? Have any improvements not long ago been manufactured on the codebase? Has this difficulty transpired ahead of below comparable circumstances? The intention is to slender down opportunities and recognize possible culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled environment. For those who suspect a certain perform or component, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code inquiries and let the effects direct you nearer to the reality.
Pay shut focus to compact information. Bugs frequently disguise in the the very least anticipated sites—just like a lacking semicolon, an off-by-a single mistake, or even a race ailment. Be comprehensive and patient, resisting Developers blog the urge to patch The problem without the need of entirely knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Lastly, hold notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Creating exams is one of the best tips on how to improve your debugging expertise and Total progress performance. Tests not only aid catch bugs early but in addition function a security Internet that offers you self esteem when earning changes for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint just the place and when a challenge occurs.
Start with device checks, which deal with unique capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where by to glimpse, appreciably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously being preset.
Following, integrate integration tests and close-to-conclusion assessments into your workflow. These support make certain that various aspects of your application function alongside one another efficiently. They’re especially useful for catching bugs that come about in sophisticated systems with many elements or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can target correcting the bug and view your examination go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, creating exams turns debugging from the disheartening guessing sport into a structured and predictable course of action—encouraging you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are much too near the code for much too extensive, cognitive exhaustion sets in. You might begin overlooking obvious errors or misreading code that you wrote just hours before. During this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different task for ten–15 minutes can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you come upon is more than just A brief setback—It can be a possibility to develop like a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, every one can instruct you something beneficial in case you make the effort to replicate and analyze what went Incorrect.
Commence by inquiring on your own a handful of key questions once the bug is resolved: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code opinions, or logging? The solutions generally expose blind spots with your workflow or comprehension and make it easier to Make more robust coding behaviors transferring ahead.
Documenting bugs can be a fantastic routine. Hold a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you discovered. As time passes, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively stay away from.
In team environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts staff effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical elements of your development journey. All things considered, a few of the most effective developers are usually not the ones who produce excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and persistence — although the payoff is large. It tends to make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.